Client-Side Pagination in LWC
You will learn the following things
- Create a new component
- Component composition
- for:each loop
- parent component to child component data passing
- Child to parent data passing using custom event
- getter and setter
- fetching data from apex
- Wire service
Video Tutorial
Code
- Create Lwc component
paginationDemo
and add the following code to the respective files.
paginationDemo.html
<template>
<lightning-card title="Pagination demo contact" icon-name="custom:custom63">
<div class="slds-var-m-around_medium">
<template if:true={visibleContacts}>
<template for:each={visibleContacts} for:item="contact">
<p key={contact.Id}>{contact.Name}</p>
</template>
</template>
<div slot="footer" class="slds-var-m-vertical_medium">
<c-pagination records={totalContacts} onupdate={updateContactHandler}></c-pagination>
</div>
</div>
</lightning-card>
<lightning-card title="Pagination demo Accounts" icon-name="custom:custom63">
<div class="slds-var-m-around_medium">
<template if:true={visibleAccounts}>
<template for:each={visibleAccounts} for:item="account">
<p key={account.Id}>{account.Name}</p>
</template>
</template>
<div slot="footer" class="slds-var-m-vertical_medium">
<c-pagination records={totalAccounts} record-size="6" onupdate={updateAccountHandler}></c-pagination>
</div>
</div>
</lightning-card>
</template>
paginationDemo.js
import { LightningElement, wire } from 'lwc';
import getContactList from '@salesforce/apex/DataController.getContactList'
import getAccountList from '@salesforce/apex/DataController.getAccountList'
export default class PaginationDemo extends LightningElement {
totalContacts
visibleContacts
totalAccounts
visibleAccounts
@wire(getContactList)
wiredContact({error, data}){
if(data){
this.totalContacts = data
console.log(this.totalContacts)
}
if(error){
console.error(error)
}
}
@wire(getAccountList)
wiredaccount({error, data}){
if(data){
this.totalAccounts = data
console.log(this.totalAccounts)
}
if(error){
console.error(error)
}
}
updateContactHandler(event){
this.visibleContacts=[...event.detail.records]
console.log(event.detail.records)
}
updateAccountHandler(event){
this.visibleAccounts=[...event.detail.records]
console.log(event.detail.records)
}
}
paginationDemo.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>49.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
</targets>
</LightningComponentBundle>
- Let's Create an child component
pagination
and add the following code to the respective file.
pagination.html
<template>
<lightning-layout>
<lightning-layout-item>
<lightning-button label="Previous"
icon-name="utility:chevronleft"
onclick={previousHandler}
disabled={disablePrevious}></lightning-button>
</lightning-layout-item>
<lightning-layout-item flexibility="grow">
<p class="slds-text-align_center">Displaying {currentPage} of {totalPage} Page</p>
</lightning-layout-item>
<lightning-layout-item>
<lightning-button label="next"
icon-name="utility:chevronright"
icon-position="right"
onclick={nextHandler}
disabled={disableNext}></lightning-button>
</lightning-layout-item>
</lightning-layout>
</template>
pagination.js
import { LightningElement, api } from 'lwc';
export default class Pagination extends LightningElement {
currentPage =1
totalRecords
@api recordSize = 5
totalPage = 0
get records(){
return this.visibleRecords
}
@api
set records(data){
if(data){
this.totalRecords = data
this.recordSize = Number(this.recordSize)
this.totalPage = Math.ceil(data.length/this.recordSize)
this.updateRecords()
}
}
get disablePrevious(){
return this.currentPage<=1
}
get disableNext(){
return this.currentPage>=this.totalPage
}
previousHandler(){
if(this.currentPage>1){
this.currentPage = this.currentPage-1
this.updateRecords()
}
}
nextHandler(){
if(this.currentPage < this.totalPage){
this.currentPage = this.currentPage+1
this.updateRecords()
}
}
updateRecords(){
const start = (this.currentPage-1)*this.recordSize
const end = this.recordSize*this.currentPage
this.visibleRecords = this.totalRecords.slice(start, end)
this.dispatchEvent(new CustomEvent('update',{
detail:{
records:this.visibleRecords
}
}))
}
}
pagination.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>49.0</apiVersion>
<isExposed>false</isExposed>
</LightningComponentBundle>
- Create an Apex class
DataController.cls
and add the following code to the file.
DataController.cls
public with sharing class DataController {
@AuraEnabled(cacheable= true)
public static List<Contact> getContactList(){
return [SELECT Id, Name FROM Contact];
}
@AuraEnabled(cacheable= true)
public static List<Account> getAccountList(){
return [SELECT Id, Name FROM Account];
}
}
Final Output
Now place your component to the record page. You will see the following output
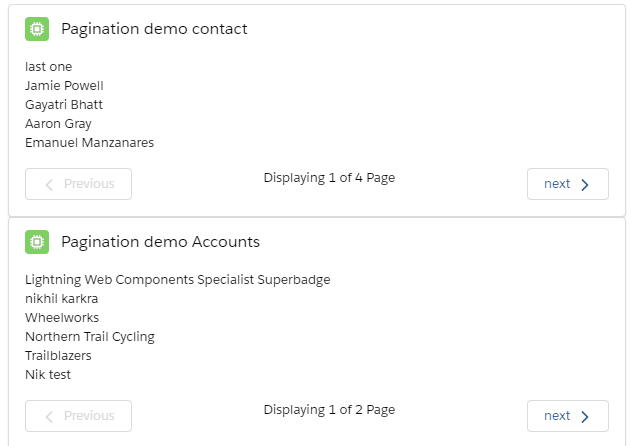