Parent to Child Communication by calling the Child method from the parent component.
In this article, we will see how we can communicate from Parent to Child Communication by calling the Child method from the parent component.
Let's understand how this works from the below fig.
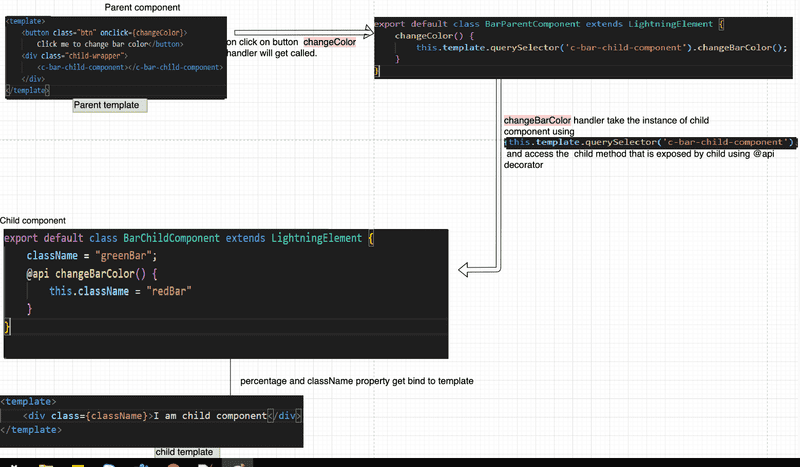
- Create two LWC Components
barParentComponent
andbarChildComponent
. - Add the code to the corresponding file from the code given below.
- In the parent component,
barParentComponent .html
we have created alightning-card
to give our component better looks and feel - Withing the
lightning-card
we have created abutton
with an event attributeonclick
that will trigger thechangeColor
handler on click of the button - Below button we are calling the child component using
c-bar-child-component
syntax. -
In
barParentComponent .js
file, we have created achangeColor
handler in which we call the following linethis.template.querySelector('c-bar-child-component').changeBarColor();
this.template.querySelector
is used to fetch the element from the DOM. In our case, we are calling the child component. It will fetch all the properties of thebarChildComponent
and from which we are accessing thechangeBarColor
method. changeBarColor
is the custom method of the child component and this method to be available on the parent we have to make it public using the@api
- In
barChildComponent
we have created one local propertyclassName
and one public methodchangeBarColor
- In the
changeBarColor
we are updating theclassName
property value - in
barChildComponent.html
based on theclassName
property value, the background color changes. - Overall, when we click the button in the parent component, it will call the
changeColor
method of child component and change the class which alter the background of the child template
On Click demo Output
After placing the component on the page, you will see the following output.
Parent component
import { LightningElement } from 'lwc';
export default class BarParentComponent extends LightningElement {
changeColor() {
this.template.querySelector('c-bar-child-component').changeBarColor();
}
}
<template>
<div class="margin-bottom-2rem">
<lightning-card title="Calling child method from parent" icon-name="custom:custom14">
<div class="slds-m-around_medium">
<div class="parent-wrapper">
<button class="btn" onclick={changeColor}>Click me to change bar color</button>
<div class="child-wrapper">
<c-bar-child-component></c-bar-child-component>
</div>
</div>
</div>
</lightning-card>
</div>
</template>
.btn {
border: none; /* Remove borders */
color: white; /* Add a text color */
padding: 14px 28px; /* Add some padding */
cursor: pointer; /* Add a pointer cursor on mouse-over */
background: #2196f3;
}
.parent-wrapper{
padding: 10px;
border: 5px solid #00a1e0;
}
.child-wrapper{
padding: 10px;
border: 2px solid #3cc2b3;
margin: 10px;
}
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__RecordPage</target>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>
Child component
import { LightningElement, api } from 'lwc';
export default class BarChildComponent extends LightningElement {
className = "greenBar";
@api changeBarColor() {
this.className = "redBar"
}
}
<template>
<div class={className}>I am child component</div>
</template>
.redBar{
background:red;
height: 50px;
margin: 10px;
color: white;
text-align: center;
line-height: 50px;
}
.greenBar{
background:green;
height: 50px;
margin: 10px;
color: white;
text-align: center;
line-height: 50px;
}
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>false</isExposed>
</LightningComponentBundle>
On Click demo Output
After placing the component on the page, you will see the following output.