Parent to Child Communication with Array/Object data
The most common data structure in any programming language is Array and objects. In this article, we will see how we can communicate from parent to child bypassing the objects and arrays.
Let's understand how parent component passes array/objects data to child component from the below fig.
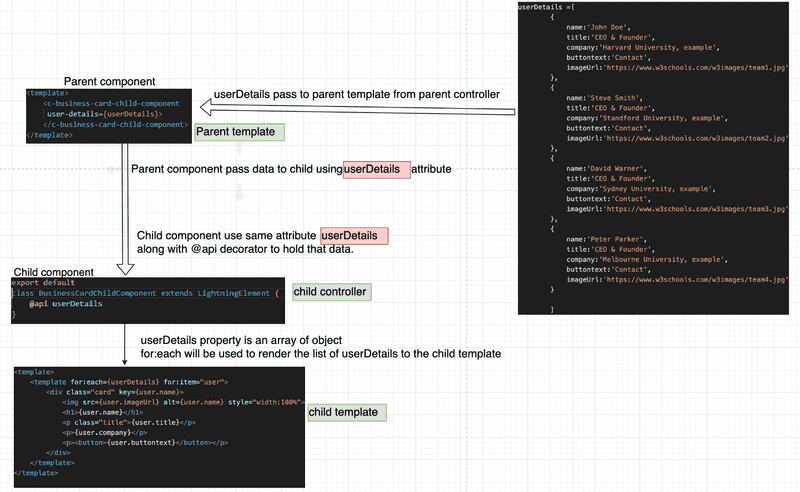
- First, we create the data to a local property in the parent component. Data can be static, or it may come from apex based on the requirement. IN our above diagram, we are using an array of objects.
- We call the child component inside the parent component and use the child component public property i.e., decorated with
@api
. - Map the parent property (
userDetails
) that has the Array of the object to the public property(user-details
) of the child component. - Once the child component load, it receives the data from the parent component using the public property, i.e., decorated by the
@api
. Once the data is available, you can use it based on your use case.
Example of Parent to Child Communication with Array/Objects data
Create a businessCardParentComponent
LWC component and add the following code to the respective file.
Parent component
import { LightningElement } from 'lwc';
export default class BusinessCardParentComponent extends LightningElement {
userDetails = [
{
name: 'John Doe',
title: 'CEO & Founder',
company: 'Harvard University, example',
buttontext: 'Contact',
imageUrl: 'https://www.w3schools.com/w3images/team1.jpg'
},
{
name: 'Steve Smith',
title: 'CEO & Founder',
company: 'Standford University, example',
buttontext: 'Contact',
imageUrl: 'https://www.w3schools.com/w3images/team2.jpg'
},
{
name: 'David Warner',
title: 'CEO & Founder',
company: 'Sydney University, example',
buttontext: 'Contact',
imageUrl: 'https://www.w3schools.com/w3images/team3.jpg'
},
{
name: 'Peter Parker',
title: 'CEO & Founder',
company: 'Melbourne University, example',
buttontext: 'Contact',
imageUrl: 'https://www.w3schools.com/w3images/team4.jpg'
}
]
}
<template>
<div class="margin-bottom-2rem">
<lightning-card title="Parent to child data communication using Array/Objects" icon-name="custom:custom14">
<div class="slds-m-around_medium">
<c-business-card-child-component user-details={userDetails}></c-business-card-child-component>
</div>
</lightning-card>
</div>
</template>
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__RecordPage</target>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>
Explanation of businessCardParentComponent
In businessCardParentComponent.js
we have initialized the local property userDetails
with an array of objects. This data can come from anywhere, either from APEX or from local stubs.
In businessCardParentComponent.html
we are composing the businessCardChildComponent
. To embed the child component in our parent component, we need to use the syntax
Note - In Lightning Web Components we should conventionally use camelCase (lower case first letter, upper case subsequent words) to name the component and kebab-case (lower case words preceded with c- and spaced with '-' minus sign) when nesting the components in a composition scenario.
Now We have to pass the data to the child component so, we need to use the attribute name that is the same as the public variable name defined in the child component (businessCardChildComponent
), i.e., userDetails
in our example.
If your child public property is camelCase as in our case userDetails
, then we need to use the attribute as user-details
in our child component calling.
Child component
Create a businessCardChildComponent
LWC component and add the following code to the respective file.
import { LightningElement, api } from 'lwc';
export default
class BusinessCardChildComponent extends LightningElement {
@api userDetails
}
<template>
<template for:each={userDetails} for:item="user">
<div class="card" key={user.name}>
<img src={user.imageUrl} alt={user.name} style="width:100%">
<h1>{user.name}</h1>
<p class="title">{user.title}</p>
<p>{user.company}</p>
<p><button>{user.buttontext}</button></p>
</div>
</template>
</template>
:host{
display: flex;
}
.card {
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2);
max-width: 300px;
margin: 10px;
text-align: center;
font-family: arial;
}
.title {
color: grey;
font-size: 18px;
}
button {
border: none;
outline: 0;
display: inline-block;
padding: 8px;
color: white;
background-color: #000;
text-align: center;
cursor: pointer;
width: 100%;
font-size: 18px;
}
a {
text-decoration: none;
font-size: 22px;
color: black;
}
button:hover, a:hover {
opacity: 0.7;
}
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>false</isExposed>
</LightningComponentBundle>
Explanation of businessCardChildComponent
In the child component businessCardChildComponent
, we have declared a property userDetails
and made it public by using the @api decorator.
In alertChildComponent.html,
we are using the for:each
loop to iterate over the data coming from the parent component. Extracting the properties like imageUrl
, name
, title
, company
, buttontextfrom the
user` object, and mapping it to the respective elements.
We have used some CSS as well to keep the design of our component decent.
on change demo Output
After placing the component on the page, you will see the following output.
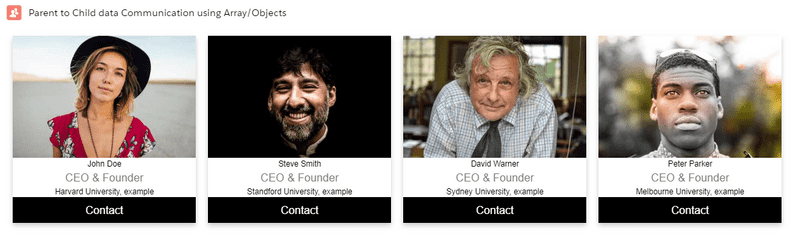