LWC IntroductionWhy Lightning Web ComponentLWC Browser supportSalesforce DX environment setupSalesforce DX project setupHello world using LWCOne-Way Data BindingTwo-Way Data Binding (@track)Conditional Renderingfor:each loopiterator loopRender multiple templateParent to Child Communication with string dataParent to Child Communication with Array/Object dataParent to Child Communication on action at parentParent to Child Communication by calling the Child method from Parent component.Child to Parent Communication by simple actionChild to Parent Communication by passing data on actionChild to Parent Communication by event bubbling
Child to Parent Communication by event bubbling
Event bubbling and capturing are two ways of event propagation in the HTML DOM API, when an event occurs in an element inside another element, and both elements have registered a handle for that event
The event propagation mode determines in which order the elements receive the event.
With bubbling, the event is first captured and handled by the innermost element and then propagated to outer elements
With capturing, the event is first captured by the outermost element and propagated to the inner elements.
The three event flow phase are illustrated in the following diagram from the W3C UIEvents specification.
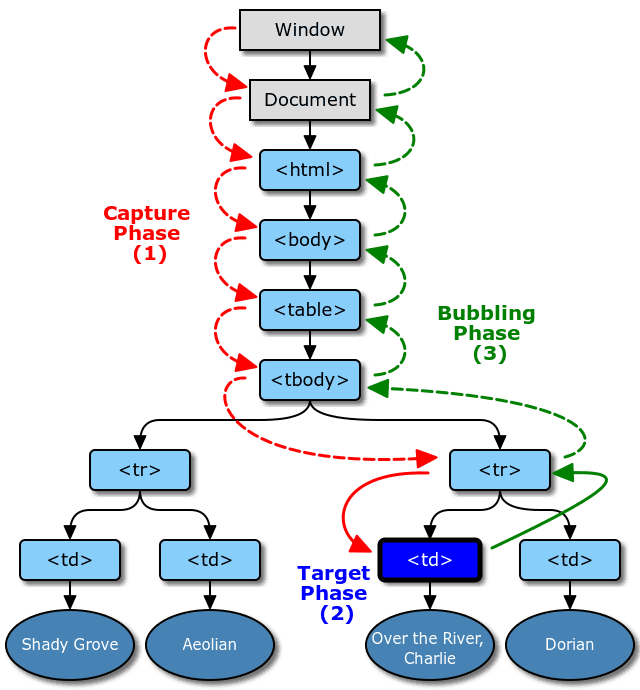
Event Bubbling Demo and explanation
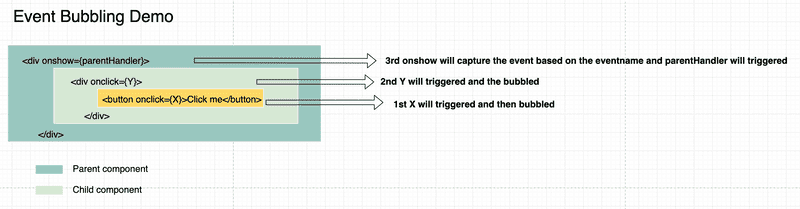
As shown above in the image
- Child component has button with event (x) and on click of that we are dispatching an event in bubbling phase
- Button is wrapped by another element on which there is another click event(Y).
- Parent element is listening to the event on div with method (Z)
- Once the button is clicked event(x) triggered and event start propogating towards upward.
- First it encountered the (y) and trigger that event and continoue the propogation
- Then it encountered the (Z) and triggerd the parent event
Event Bubbling in LWC
- Create two LWC components
notifyParentComponent
andnotifyChildComponent
. - Create Css file in both the components.
- Add the code to the corresponding file from the code given below.
notifyParentComponent
notifyParentComponent.js
import { LightningElement } from 'lwc';
export default class NotifyParentComponent extends LightningElement {
showNotification = false;
showHandler() {
this.showNotification = true;
}
}
notifyParentComponent.html
<template>
<lightning-card title="Event with Bubbling" icon-name="custom:custom14">
<div class="slds-m-around_medium">
<div class="parent-section" onshow={showHandler}>
<p>I am parent component</p>
<template if:true={showNotification}>
<div class="notificationbox">
<span>Notification from Child. Event bubbled successfully!</span>
</div>
</template>
<c-notify-child-component></c-notify-child-component>
</div>
</div>
</lightning-card>
</template>
notifyParentComponent.css
.notificationbox {
background-color: #43a047;
color: #fff;
display: flex;
padding: 16px 16px;
flex-grow: 1;
flex-wrap: wrap;
align-items: center;
border-radius: 4px;
flex-grow: initial;
max-width: 80%;
margin-bottom: 1rem;
}
.parent-section {
border: 5px solid black;
padding: 2rem;
}
notifyParentComponent.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__RecordPage</target>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>
- In the parent component,
notifyParentComponent.html
we have created alightning-card
to give our component better looks and feel - Withing the
lightning-card
we have adiv
withparent-section
class in which we are listening to the custsom eventonshow
that is call ing theshowHandler
- Within
parent-section
, we have two main part one is notification box that is only visibile whenshowNotification
prooperty become true and second part is the child componentc-notify-child-component
- In
notifyParentComponent.js
, we have one local propertyshowNotification
that is initialized withfalse
andshowHandler
method that will update theshowNotification
value
notifyChildComponent.js
notifyChildComponent
import { LightningElement } from 'lwc';
export default class NotifyChildComponent extends LightningElement {
showChildNotification = false;
childHandler() {
this.showChildNotification = true;
}
showNotifyParentHandler(event) {
event.preventDefault();
const selectEvent = new CustomEvent('show', {
bubbles: true
});
this.dispatchEvent(selectEvent);
}
}
notifyChildComponent.html
<template>
<div class="child-section">
<p>I am child component</p>
<template if:true={showChildNotification}>
<div class="notificationbox">
<span>Notification in Child component. Event bubbled till parent
element in same component</span>
</div>
</template>
<div class="content" onclick={childHandler}>
<button class="btn" onclick={showNotifyParentHandler}>
Click me to send notification to parent component using event bubbling
</button>
</div>
</div>
</template>
notifyChildComponent.css
.notificationbox {
background-color: #ffa000;
color: #fff;
display: flex;
padding: 16px 16px;
flex-grow: 1;
flex-wrap: wrap;
align-items: center;
border-radius: 4px;
flex-grow: initial;
max-width: 80%;
margin-bottom: 1rem;
}
.child-section {
border: 5px solid red;
padding: 2rem;
}
.btn {
border: none;
color: white;
padding: 14px 28px;
font-size: 16px;
cursor: pointer;
background-color: #01344e;
}
.content {
display: contents;
}
notifyChildComponent.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>48.0</apiVersion>
<isExposed>false</isExposed>
</LightningComponentBundle>
- In
notifyChildComponent.html
, we have notificationbox that will be visible only when the value of theshowChildNotification
property become true - Also, in template we have content section which has a onclick event that calls the
childhandler
. In between content template we have a button that too has onclick event which calls theshowNotifyParentHandler
method. - So when we click on the inner element that is button. Event start bubbling and it automatically call the
childhandler
and after that it will go to parent component and trigger it'sshowHandler
as well.
Output
After placing the component on the page, you will see the following output.